Tips for .NET Dev, Part I
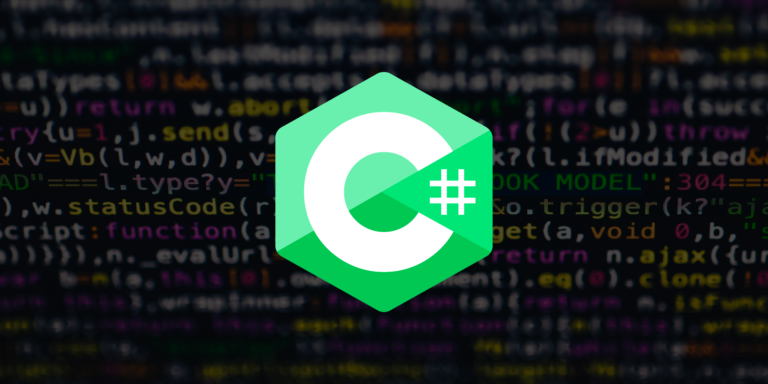
IT Tips & Insights: A Softensity Senior Software Developer shares tips for C# and general programming in this multi-part series.
By Gabriel Cordeiro, Senior Software Developer
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.”
― Martin Fowler
When I first saw this sentence by Martin Fowler, my mind was blown. I understood something very simple: We are writing code for people. We’re writing a text or a book with many chapters, and if we organize it very well, it will be easier to understand. That is the point of this series: Keep it simple, organized, and small. With that in mind, let’s start with the first tip to make prettier code.
Object Initializers
The first tip is a feature from C# 3.0 that makes your code readable, easy to add elements to, and simple. Basically, you can use Object Initializers to assign values to any accessible fields or properties of any object in C#. That means you can use it for classes, arrays, lists, even for anonymous types. Let’s take a look at some examples.
What you may want to avoid:
What you can do instead:
I’d recommend it for Anemic, DTO, or POCO classes. It doesn’t substitute constructors, but it can work together with constructors when you have optional parameters that don’t belong to the constructor.
Create Methods/Functions
When you start to learn concepts of programming like SOLID, clean code, and others, there are two key points: “Names are important” and “Keep it simple.” Let’s look at how to make something prettier and simpler using Functions — aka, as Methods.
Methods are very important and common, but sometimes we forget to use them for simple things. When I was in college, I learned structural programming, and I noticed that sometimes people (including me) think more structurally. For example, writing down all of the steps for an algorithm can make everything hard to understand — and necessary to read it again and again.
When we write methods, we have names, and names make everything more understandable. Let’s see some examples, shall we?
The problem: We need to create a function that reverses a sentence and let the first letter for each word be uppercase.
What a mess right? What is happening there?
- A function receiving a text as a parameter and reversing it with all letters lowercase
- Condition for text with more than one word
- For each word add uppercase to the first letter
- Returning the reversed text
There are many things that can be improved, but the goal here is how we can make it prettier using methods. Let’s see it:
Easier, right? Basically, I created a method for each step, making everything more readable, testable and easy to change. I created some extension methods to help me to write fewer lines. If you don’t know about extensions methods, you can read about them here: Extension Methods – C# Programming Guide | Microsoft Docs
About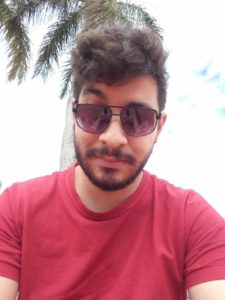
Hey there, I’m Gabriel. I’m 24 years old and I’ve been a software engineer for almost 7 years. Currently, I’m a Senior Software Developer at Softensity. Most of my experience has been coding with C# from Microsoft, and I’ve learned a lot of cool things in this amazing language, so I thought I’d share some tips, both for C#, and programming in general.